Why to use
-
More quick, more fast
-
Apply to interval summation, so if you use array ( ) or prefix-summation ( modification , query of interval summation ), so when the is very big, it will be slow.
Binary Indexed Tree
Operations
-
( ) add to the values of elements
-
( ) calculate the sum of range
Basic Properties
-
Firstly, BIT is a kind of array, also a kind of tree.
-
Secondly, BIT stored in memory as an array.
-
Thirdly, for two array index , if ( k is the number of 0 of ), so we define is a couple father and son on tree. In this, y is the father, x is the son.
Store
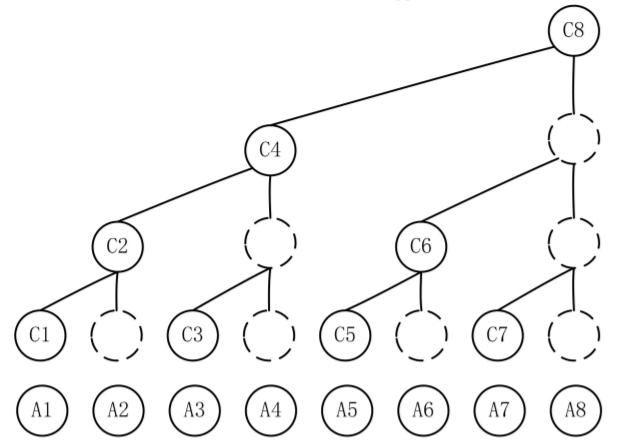
Like this. shows son-tree's leaves summation.
So:
=
= +
=
= + + +
=
= +
=
= + + + + + + +
If we change the array- to binary:
(1 = 001)
(2 = 010)
(3 = 011)
(4 = 100)
(5 = 101)
(6 = 110)
(7 = 111)
(8 = 1000)
We could find out that: ( is the binary of the successive from low-digit to high-digit)
BIT Query Intercal Summation
eqauls
-
So, we could add some elements of array to get the intercal summation.
-
lowbit (i)
Get the value of . is the binary of the successive from low-digit to high-digit.
-
While the is more bigger, BIT's goodness is more clearly.
-
We can "minus" the at the end of the binary of , that means we need to minus .
inline int sum ( int x ) {
int ans = 0 ;
while ( x > 0 ) {
ans += c[x] ;
x -= lowbit (x) ;
}
return ans ;
}
BIT Single Point Modification
- While we change array , we need to update array . It will be a reverse process of
sum(i)
.
inline void add ( int x , int d ) {
while ( x <= n ) {
c[x] += d ;
x += lowbit (x) ;
}
}
Time Complexity
Modification & Summation
-
In
add ( x , d )
, we need add to each interval containing . As same as insum (x)
, we need to count the sum of intervals. -
In
lowbit (x)
, every time you deal with an interval, you need alowbit (x)
function. So it's too.
Lowbit Function
- Simple version of
lowbit (x)
function:
inline int lowbit ( int x ) {
int ans = 1 ;
while ( x % 2 == 0 ) {
x /= 2 ;
ans *= 2 ;
}
return ans ;
}
Summary
So, if you use the simple version of lowbit (x)
function, the sum time complexity will be
More Quick Of Lowbit
-
We can use complement code to calculate it.
-
Code:
inline int lowbit ( int x ) {
return x & -x ;
}
- So now the time complexity is , memory complexity .
BIT Extention
Interval Modification, Single Point Query
-
( , ) add to the elements in interval .
-
( print ) query the value of element .
Single Point Modification & Interval Modification
-
We can use difference to solve this problem, turn what array element wants to the value of . Solution like the down text.
-
We use array to:
-
If eqauls ,
-
If ,
-
We get array use BIT on array , then
-
Single Point Query & Interval Summation
-
Like last page, it also can use difference to solve the problem.
-
We don't need every elements add on a . You see, , when we add on left, we just need to add only one k on right to keep it's balance.
-
So, if we want to add k on the elements of to , we should: .